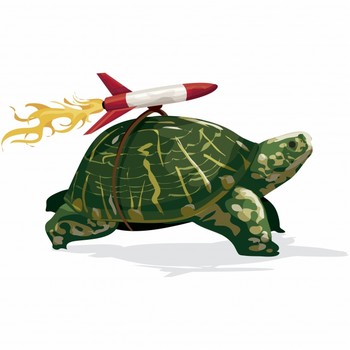
So, to stop it running too fast: First, define a variable of type "long" at the start of your program for how long you would like the program to take over each game loop. In the example below, I've chosen the name "LOOP_DELAY" for this var, and I usually give it a value of around 25. You'll probably want to tweak this amount. Also, declare another long called "start_time".
Anyway, at the start of your "game loop", write something like:-
start_time = System.getCurrentTimeMillis();
This will store the current time when the computer started the game loop. Finally, at the end of your game loop, write something like:-
long wait = LOOP_DELAY - System.currentTimeMillis() + start_time;
Thread.sleep(wait);
(You'll need to wrap this in a try/catch BTW). This bit of code will make the program pause for a certain amount of time at the end of each game loop, that time being the number of milliseconds you wanted it to take over each game loop, minus the amount of time it took to actually process the code in your game loop. This will cause each game loop to take a consistent amount of time, assuming that the time it takes to process the code in the game loop isn't longer than the time you want it to wait for!
No comments:
Post a Comment